In most mobile applications, you’re going to be presenting your users a list of something. Most of the time, it’s not as simple as an array of strings; your data may be stored away in a local Sqlite database, or perhaps behind a RESTful API. In any case, we’ll be taking a look at what it takes to begin with a simple ListActivity that can be easily updated later on.
Create a new Android Project (1.1+) and name the activity MyListActivity (or whatever you prefer.) Run the app to ensure you’re working off a valid clean slate.
Next, modify your activity (MyListActivity) to extend the Android class ListActivity and adjust your code to look like the following:
So far, so good. We have a method to create our list adapter, so essentially, our onCreate() method is done. Let’s move on to adapters.
Adapters basically glue a collection of objects to an activity, usually by providing a mapping or instructions on how to render each object in the list for the activity. In our case, we have a simple list of strings, so our adapter is pretty straightforward (and in fact, is already provided by Android: ArrayAdapter)
Modify your createAdapter() method to look like the following:
This encapsulates everything we need to get an adapter up and running. Typically here we may make a call-out to data, which may be a Sqlite query or a RESTful GET request, but for now we’re using simple strings. We create an ArrayAdapter and specify three things: Context, Layout, and Data.
The Context is simply where the list came from. In our app it’s simple, but sometimes it can get a little complex when you’re passing intents back and forth across activities, so this is used to keep a reference to the owner activity at all times.
The Layout is where the data will be rendered (and how it will look.) We’re using a built-in Android layout for our needs (simple_list_item_1).
The Data is what will be rendered, and is a simple list of strings.
Final result should look like the following:
Run the app, and you should see something like this:
And there you have a nice introduction to a ListActivity that can be expanded upon by focusing on the createAdapter() method. In Part 2, we’ll explore using more complex Object lists and custom Adapters, as well as a brief introduction to the layout files. Thanks for reading!
Create a new Android Project (1.1+) and name the activity MyListActivity (or whatever you prefer.) Run the app to ensure you’re working off a valid clean slate.
Next, modify your activity (MyListActivity) to extend the Android class ListActivity and adjust your code to look like the following:
package com.learnandroid.listviewtutorial.simple; import android.app.ListActivity; import android.os.Bundle; import android.widget.ListAdapter; public class MainListView extends ListActivity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); ListAdapter adapter = createAdapter(); setListAdapter(adapter); } /** * Creates and returns a list adapter for the current list activity * @return */ protected ListAdapter createAdapter() { return null; } }
Adapters basically glue a collection of objects to an activity, usually by providing a mapping or instructions on how to render each object in the list for the activity. In our case, we have a simple list of strings, so our adapter is pretty straightforward (and in fact, is already provided by Android: ArrayAdapter)
Modify your createAdapter() method to look like the following:
/** * Creates and returns a list adapter for the current list activity * @return */ protected ListAdapter createAdapter() { // Create some mock data String[] testValues = new String[] { "Test1", "Test2", "Test3" }; // Create a simple array adapter (of type string) with the test values ListAdapter adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, testValues); return adapter; }
The Context is simply where the list came from. In our app it’s simple, but sometimes it can get a little complex when you’re passing intents back and forth across activities, so this is used to keep a reference to the owner activity at all times.
The Layout is where the data will be rendered (and how it will look.) We’re using a built-in Android layout for our needs (simple_list_item_1).
The Data is what will be rendered, and is a simple list of strings.
Final result should look like the following:
package com.learnandroid.listviewtutorial.simple; import android.app.ListActivity; import android.os.Bundle; import android.widget.ArrayAdapter; import android.widget.ListAdapter; public class MainListView extends ListActivity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); ListAdapter adapter = createAdapter(); setListAdapter(adapter); } /** * Creates and returns a list adapter for the current list activity * @return */ protected ListAdapter createAdapter() { // Create some mock data String[] testValues = new String[] { "Test1", "Test2", "Test3" }; // Create a simple array adapter (of type string) with the test values ListAdapter adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, testValues); return adapter; } }
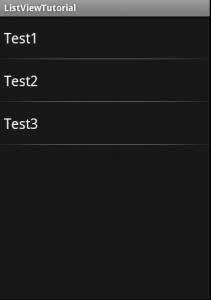
A screen shot of an example list of strings
Samsun
ReplyDeleteUrfa
Erzincan
Mersin
Kayseri
0DPW
918AD
ReplyDeleteDiyarbakır Lojistik
Antalya Parça Eşya Taşıma
Karaman Evden Eve Nakliyat
Karabük Parça Eşya Taşıma
Mardin Evden Eve Nakliyat
356FF
ReplyDeleteHakkari Parça Eşya Taşıma
İzmir Evden Eve Nakliyat
Kars Evden Eve Nakliyat
Tekirdağ Lojistik
Ordu Parça Eşya Taşıma
23B76
ReplyDeleteSiirt Parça Eşya Taşıma
Giresun Parça Eşya Taşıma
Niğde Parça Eşya Taşıma
Manisa Parça Eşya Taşıma
Antalya Lojistik
4151C
ReplyDeleteUşak Şehirler Arası Nakliyat
Bartın Evden Eve Nakliyat
Ardahan Evden Eve Nakliyat
Kastamonu Lojistik
Aksaray Şehir İçi Nakliyat
Osmaniye Şehir İçi Nakliyat
Aydın Evden Eve Nakliyat
Kırşehir Şehir İçi Nakliyat
Aydın Lojistik
04302
ReplyDeleteProbit Güvenilir mi
Çerkezköy Yol Yardım
Huobi Güvenilir mi
İzmir Lojistik
Ünye Parke Ustası
Adıyaman Evden Eve Nakliyat
Bartın Şehirler Arası Nakliyat
Aksaray Şehir İçi Nakliyat
Kütahya Parça Eşya Taşıma
2F7E4
ReplyDeleteKırklareli Evden Eve Nakliyat
Sivas Evden Eve Nakliyat
testosterone enanthate
Tokat Evden Eve Nakliyat
Maraş Evden Eve Nakliyat
Muş Evden Eve Nakliyat
Kütahya Evden Eve Nakliyat
order turinabol
sarms
96FB6
ReplyDelete%20 binance referans kodu
39053
ReplyDeletereferans
B74AF
ReplyDeletekırşehir mobil sohbet et
urfa rastgele sohbet odaları
ordu parasız görüntülü sohbet uygulamaları
kayseri bedava sohbet uygulamaları
artvin canlı sohbet sitesi
rastgele canlı sohbet
yalova görüntülü sohbet siteleri
rize bedava görüntülü sohbet
bedava sohbet siteleri
D7FBF
ReplyDeleteKırıkkale Kadınlarla Rastgele Sohbet
bursa görüntülü sohbet odaları
sohbet uygulamaları
karabük canlı görüntülü sohbet
ardahan canlı sohbet bedava
elazığ görüntülü sohbet siteleri
Muğla Görüntülü Sohbet Canlı
canlı sohbet et
Adıyaman Ücretsiz Sohbet Uygulaması
0C4A6
ReplyDeleteburdur ücretsiz sohbet uygulaması
çanakkale en iyi sesli sohbet uygulamaları
düzce rastgele sohbet
kilis bedava sohbet
isparta canli goruntulu sohbet siteleri
giresun telefonda kadınlarla sohbet
Antep Telefonda Kadınlarla Sohbet
bayburt en iyi ücretsiz görüntülü sohbet siteleri
aydın sohbet
C5619
ReplyDeletetamamen ücretsiz sohbet siteleri
gümüşhane ucretsiz sohbet
kilis goruntulu sohbet
Bolu Rastgele Görüntülü Sohbet Uygulamaları
Düzce Yabancı Sohbet
yalova bedava sohbet siteleri
ısparta görüntülü sohbet kadınlarla
Düzce Mobil Sohbet Odaları
kütahya sesli görüntülü sohbet
4180F
ReplyDeleteKastamonu Rastgele Sohbet Siteleri
tunceli sohbet chat
telefonda rastgele sohbet
Ağrı Görüntülü Sohbet Siteleri
Izmir Mobil Sohbet Chat
rastgele sohbet odaları
canli sohbet
canlı ücretsiz sohbet
manisa ucretsiz sohbet
FBEDF
ReplyDeleteYeni Çıkacak Coin Nasıl Alınır
Binance Borsası Güvenilir mi
Onlyfans Beğeni Satın Al
Parasız Görüntülü Sohbet
Binance Referans Kodu
Tiktok Beğeni Satın Al
Facebook Sayfa Beğeni Hilesi
Azero Coin Hangi Borsada
Referans Kimliği Nedir